Our AI-powered language translator makes communication across borders easier than ever. Whether you're shopping online in a foreign language, learning a new language, or traveling, our tool provides seamless translations for texts, signs, and customer support inquiries. Built with advanced AI technology, the translator can handle multiple languages, improving efficiency and breaking down language barriers for businesses and individuals alike.
Technologies Used
- Python
- Googletrans
- ipywidgets
- IPython
In this comprehensive guide, we will walk you through building your own AI-powered Language Translator from scratch. The process includes setting up the environment, integrating the Google Translate API, creating the translation function, and building an interactive user interface with widgets. With Python, Googletrans, and ipywidgets, you'll create a robust system capable of translating text into multiple languages. Plus, you can follow along with a detailed YouTube video walkthrough that visually guides you through each step.
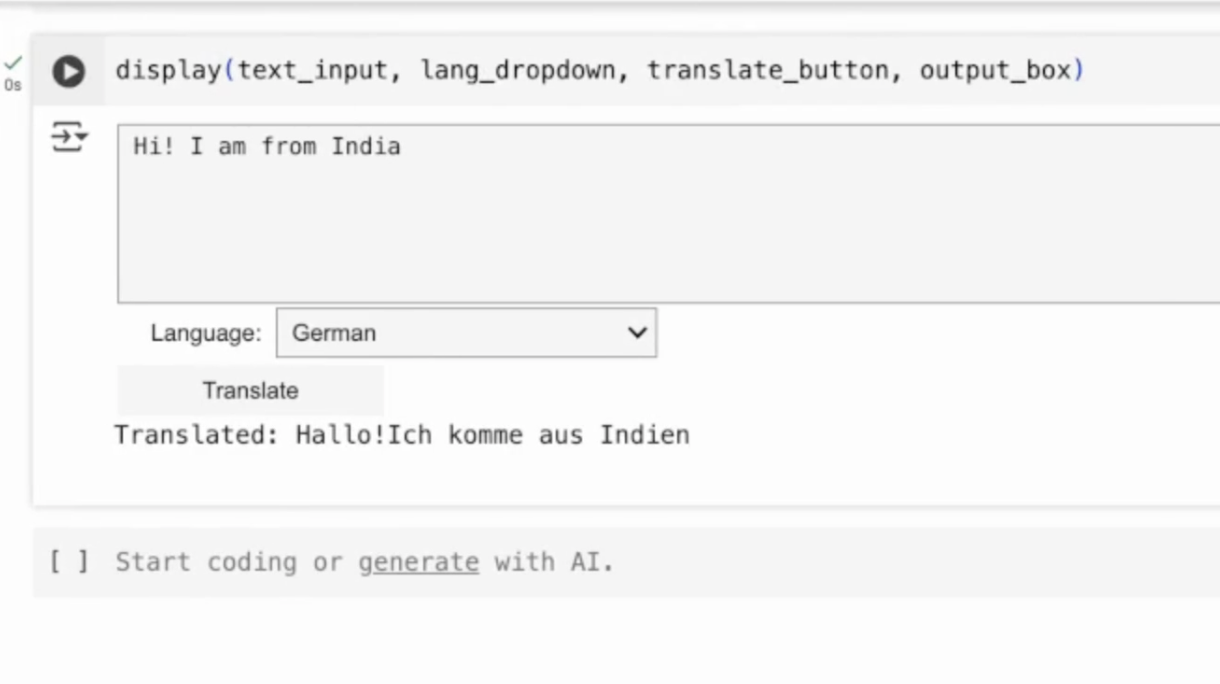
Why Build This AI?
The AI has several real-world uses, such as:
- Real-Time Communication Across Borders
- Learning New Languages Made Easy
- Customer Support Made Efficient
- Marketing & Advertising – Audience reaction analysis, ad effectiveness testing
- Travel & Tourism Made Easier
Let's Build It
!pip install googletrans==4.0.0-rc1
The following code demonstrates how to use the googletrans
library to translate text:
- Importing the Translator: The
Translator
class is imported from thegoogletrans
library. - Initializing the Translator: The
translator
object is created using theTranslator()
method. This object will be used to perform translations. - Translate Function:
- The function
translate_text(text, target_lang)
takes two parameters:text
(the text to be translated) andtarget_lang
(the language code for the target language). - The function uses
translator.translate()
to translate the text into the target language. Thedest=target_lang
specifies the desired language for translation. - The translated text is returned using
translated.text
.
- The function
- Test the Translation: In the test section, the text "Hello, how are you?" is translated to German (
de
) using the function, and the result is printed to the console.
This code provides a simple example of how to use the googletrans
library to translate text dynamically.
from googletrans import Translator
# Initialize Translator
translator = Translator()
# Function to translate text
def translate_text(text, target_lang):
translated = translator.translate(text, dest=target_lang)
return translated.text
# Test it
text = "Hello, how are you?"
target_lang = "de" # Spanish
print("Translated:", translate_text(text, target_lang))
This code demonstrates how to build an interactive translation application using ipywidgets
and IPython.display
:
- Importing Libraries:
ipywidgets
is imported aswidgets
, which allows you to create interactive UI elements such as text areas, buttons, and dropdowns.IPython.display
is imported to use thedisplay
function, which is used to display widgets in a Jupyter notebook environment.
- Creating the Text Input:
- A
Textarea
widget is created for users to input the text they want to translate. It is styled with a width of 100% and a height of 100px. A placeholder text is displayed to prompt the user to enter text.
- A
- Creating the Language Dropdown:
- A
Dropdown
widget is created to allow the user to select a target language for translation. The options include Spanish, French, German, Hindi, and Chinese, each paired with its respective language code.
- A
- Creating the Translate Button:
- A
Button
widget is created with the label "Translate." This button will trigger the translation process when clicked.
- A
- Creating the Output Box:
- An
Output
widget is created to display the translated text after the translation is performed.
- An
- Function for Button Click Event:
- The function
on_translate_clicked(b)
is triggered when the "Translate" button is clicked. Inside the function: output_box.clear_output()
clears any previous output from the output box.translate_text(text_input.value, lang_dropdown.value)
is called to translate the text input, using the selected language from the dropdown.- The translated text is printed in the output box using
print("Translated:", translated_text)
.
- The function
- Connecting Button Click to the Function:
translate_button.on_click(on_translate_clicked)
binds the button click event to theon_translate_clicked
function, so the translation is triggered when the button is clicked.
- Displaying the UI:
display()
is called to render the text input, language dropdown, translate button, and output box in the notebook so the user can interact with them.
This code creates an interactive translation interface in a Jupyter Notebook, allowing users to input text, select a language, click "Translate," and view the translated result.
import ipywidgets as widgets
from IPython.display import display
# Text Input
text_input = widgets.Textarea(
placeholder="Enter text to translate...",
layout=widgets.Layout(width="100%", height="100px")
)
# Language Dropdown
lang_dropdown = widgets.Dropdown(
options=[("Spanish", "es"), ("French", "fr"), ("German", "de"), ("Hindi", "hi"), ("Chinese", "zh-cn")],
description="Language:"
)
# Translate Button
translate_button = widgets.Button(description="Translate")
# Output Box
output_box = widgets.Output()
# Function to translate on button click
def on_translate_clicked(b):
output_box.clear_output()
translated_text = translate_text(text_input.value, lang_dropdown.value)
with output_box:
print("Translated:", translated_text)
translate_button.on_click(on_translate_clicked)
# Display UI
display(text_input, lang_dropdown, translate_button, output_box)