AI - License Plate Recognition
License Plate Recognition (LPR) is a cutting-edge AI technology that enables automatic detection of vehicle number plates from images. With this system, you can upload an image of any vehicle, and the AI will identify and extract the number plate information.
Technologies Used
- Python
- OpenCV
- Tesseract OCR
- NumPy & Matplotlib
In this comprehensive guide, we will show you how to build your own License Plate Recognition system from scratch. The process includes setting up the environment, processing images, training the model, and integrating the AI to detect number plates from uploaded vehicle images. With the power of Python, OpenCV, and deep learning, you’ll have a fully functional LPR system capable of reading license plates with high accuracy. Plus, you can check out the detailed YouTube video walkthrough to guide you through each step visually.
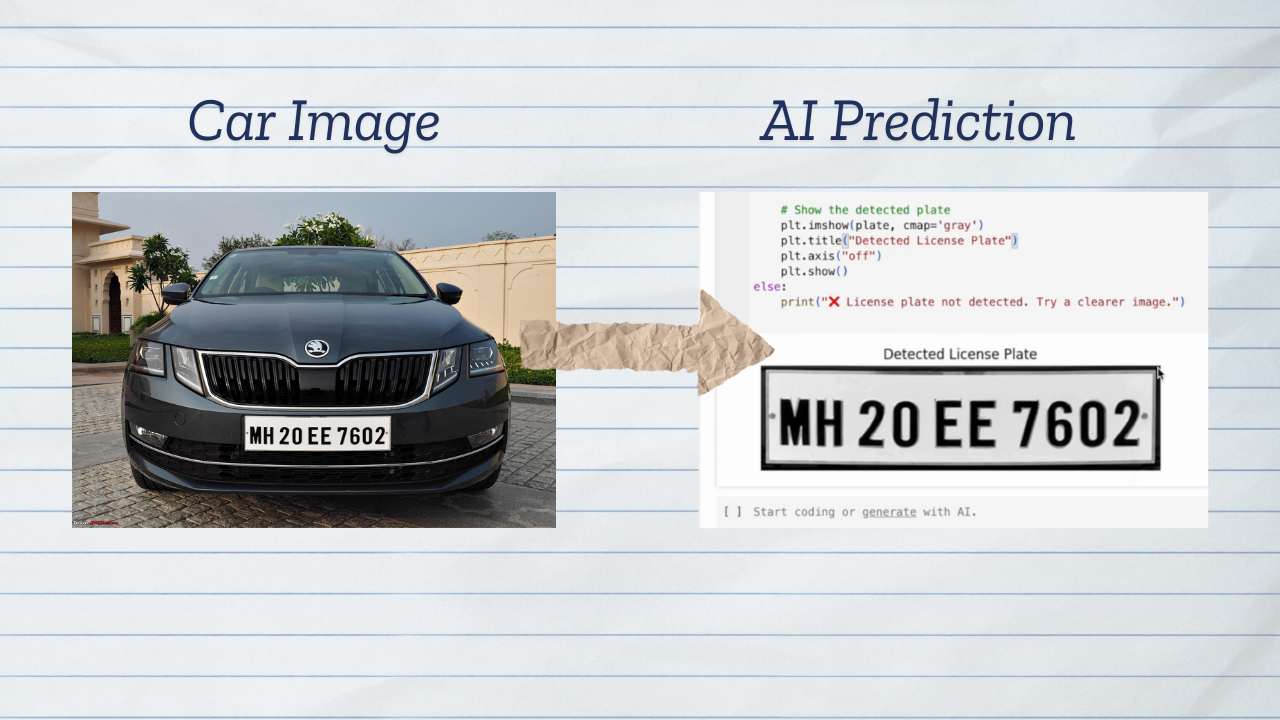
Why Build This AI?
The AI has several real-world uses, such as:
- Traffic Law Enforcement: Automates vehicle tracking and fine issuance.
- Parking Management: Logs vehicle entries/exits for smart parking systems.
- Security and Surveillance: Detects unauthorized or suspicious vehicles.
- Automated Toll Collection: Enhances toll payment efficiency through plate recognition.
Let's Build It
Installing Dependencies
Tesseract-OCR Installation
Command: !apt-get install -y tesseract-ocr
- Installs Tesseract OCR, an open-source Optical Character Recognition engine.
- Required for extracting text (like license plate numbers) from images.
Python Library Installations
Command: !pip install pytesseract opencv-python numpy matplotlib
- pytesseract: A Python wrapper for Tesseract OCR, allowing interaction with the OCR engine.
- opencv-python: Provides image processing tools like grayscale conversion, edge detection, and contour detection.
- numpy: A powerful numerical library used for handling image data as arrays.
- matplotlib: A visualization library used to display images and processing steps.
!apt-get install -y tesseract-ocr
!pip install pytesseract opencv-python numpy matplotlib
Uploading an Image
We use Google Colab's files.upload() to manually upload an image from the computer. The uploaded file's name is extracted for further processing.
from google.colab import files
# Upload the file manually from your computer
uploaded = files.upload() # This will open a file upload dialog
# Get the uploaded file name
image_path = list(uploaded.keys())[0]
print(f"✅ Uploaded file: {image_path}")
Loading and Displaying the Image
import cv2
import matplotlib.pyplot as plt
# Read the uploaded image
image = cv2.imread(image_path)
# Check if image is loaded successfully
if image is None:
print("❌ Error: Image not loaded! Check the file format and try again.")
else:
print("✅ Image loaded successfully.")
# Convert BGR (OpenCV default) to RGB for Matplotlib display
image_rgb = cv2.cvtColor(image, cv2.COLOR_BGR2RGB)
# Show the image
plt.imshow(image_rgb)
plt.axis("off")
plt.title("Uploaded Image")
plt.show()
Converting to Grayscale
The image is converted to grayscale to simplify processing and improve edge detection. It is then displayed using Matplotlib.
# Convert to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Show the grayscale image
plt.imshow(gray, cmap='gray')
plt.axis("off")
plt.title("Grayscale Image")
plt.show()
Noise Reduction & Edge Detection
A bilateral filter is applied to reduce noise while preserving edges. Then, Canny edge detection is used to highlight the contours in the image.
# Apply bilateral filter to remove noise
filtered = cv2.bilateralFilter(gray, 13, 75, 75)
# Apply Canny edge detection
edges = cv2.Canny(filtered, 50, 200)
# Show the edge-detected image
plt.imshow(edges, cmap='gray')
plt.axis("off")
plt.title("Edge Detection")
plt.show()
License Plate Detection
Finding Shapes in the Image
- It looks for objects (contours) in the image that have sharp edges.
- It keeps only the 10 biggest shapes, assuming one of them is the license plate.
Filtering the Best Match
- It checks the size and shape of each object.
- A license plate is usually a rectangle with a specific width-to-height ratio.
- The best matching shape is selected based on its size and proportions.
Extracting the License Plate
- If a good match is found, it cuts that part of the image.
- A small padding is added to improve recognition.
- Finally, the license plate area is shown.
Handling Errors
- If no suitable shape is found, it displays an error message.
import numpy as np
contours, _ = cv2.findContours(edges, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
contours = sorted(contours, key=cv2.contourArea, reverse=True)[:10] # Top 10 largest contours
plate = None
best_candidate = None
best_score = 0 # Track best match
for c in contours:
x, y, w, h = cv2.boundingRect(c)
aspect_ratio = w / h
area = w * h # Calculate area
# 🔹 Refined filtering: Ensuring realistic plate dimensions
if 2000 < area < 50000 and 2 < aspect_ratio < 5:
score = area / (w * h) # Confidence score based on area
if score > best_score:
best_score = score
best_candidate = (x, y, w, h) # Store best plate candidate
# 🔹 Extract the best candidate plate
if best_candidate:
x, y, w, h = best_candidate
# Add padding for better OCR performance
padding = 5
x, y = max(x - padding, 0), max(y - padding, 0)
w, h = min(w + 2 * padding, gray.shape[1] - x), min(h + 2 * padding, gray.shape[0] - y)
plate = gray[y:y+h, x:x+w] # Extract license plate region
# Show the detected plate
plt.imshow(plate, cmap='gray')
plt.title("Detected License Plate")
plt.axis("off")
plt.show()
else:
print("❌ License plate not detected. Try a clearer image.")