In this project, we leverage cutting-edge AI technology to detect a wide range of objects, including people, cars, dogs, cats, airplanes, and traffic lights. Using YOLOv5, a powerful pre-trained object detection model, combined with PyTorch, this system can accurately identify various objects in images. The best part is that it runs seamlessly on Google Colab, making it easy for anyone to implement and use.
Technologies Used
- Python
- YOLOv5
- PyTorch
- Pandas
In this comprehensive guide, we will show you how to build your own AI-based object detection system from scratch. The process includes setting up the environment, processing images, loading the YOLOv5 pretrained model, and detecting various objects from uploaded images. With the power of Python, PyTorch, and deep learning, you'll create a robust system capable of identifying objects like people, cars, dogs, and more. Plus, you can follow along with a detailed YouTube video walkthrough that will visually guide you through each step.
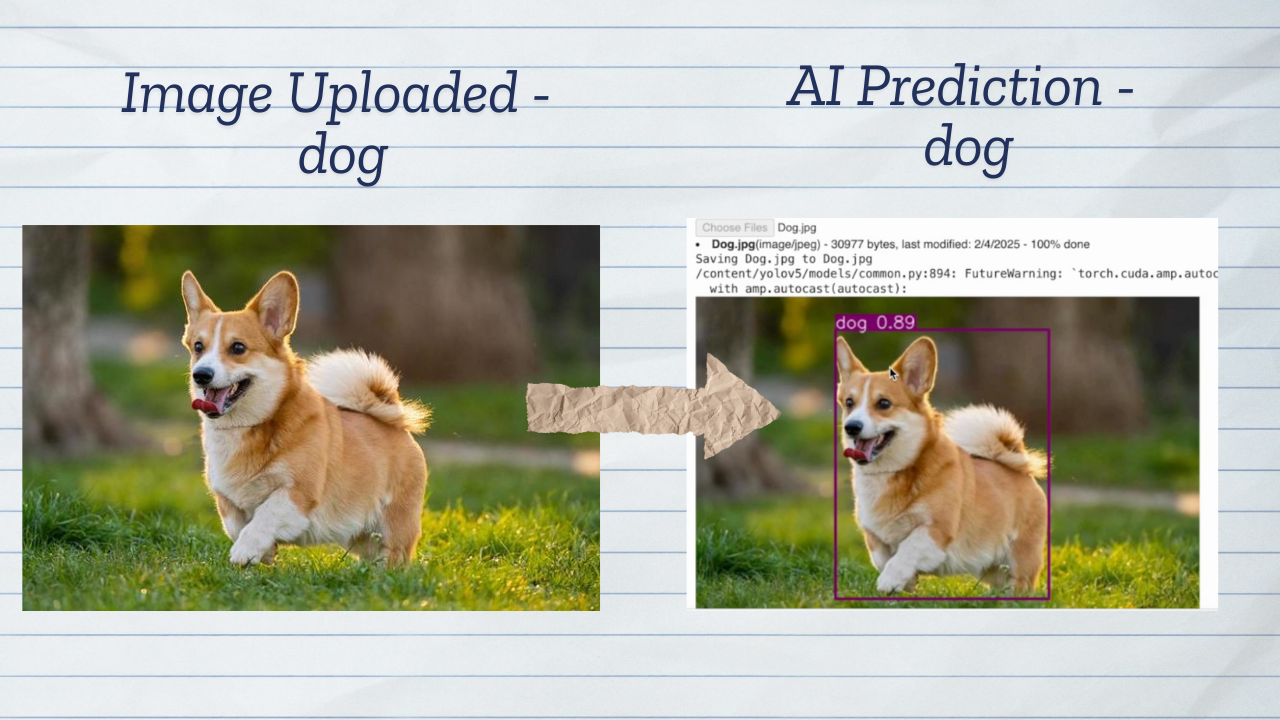
Why Build This AI?
The AI has several real-world uses, such as:
- Self-Driving Cars : Detects pedestrians, traffic lights, and other vehicles
- Security & Surveillance : Detects people or suspicious objects in CCTV footage.
- Retail & Inventory : Tracks objects in stores or warehouses.
- Medical Imaging : Can be fine-tuned to detect medical conditions.
- Sports Analytics : Tracks players and objects like footballs.
Let's Build It
Building the Code
- Cloning YOLOv5 repo: Downloads necessary files.
- Changing directory: Moves into the YOLOv5 folder.
- Installing dependencies: Ensures we have required libraries.
!git clone https://github.com/ultralytics/yolov5.git
%cd yolov5
!pip install -r requirements.txt
- Importing YOLOv5 and Torch: Required for deep learning.
- Loading Pretrained Model: Uses YOLOv5s (small variant) for object detection.
from yolov5 import detect
import torch
# Load the YOLOv5 model
model = torch.hub.load('ultralytics/yolov5', 'yolov5s', pretrained=True)
- Uploads an image from the user: Allows the user to upload an image for detection.
- Runs inference using YOLOv5: Processes the uploaded image to detect objects.
- Extracts object names and displays detection results: Displays the objects detected in the image.
# Upload an image
from google.colab import files
uploaded = files.upload()
# Get the file name
image_path = list(uploaded.keys())[0]
# Run inference
results = model(image_path)
# Extract detected object names
detected_objects = results.pandas().xyxy[0]['name'].tolist()
# Show detection results
results.show()