AI - Story Generator
In this comprehensive guide, we will walk you through building your own AI Story Generator from scratch. The process includes setting up the environment, loading a pre-trained GPT-2 model, creating a function to generate stories, and deploying an interactive web interface using Gradio. With Python, Hugging Face Transformers, and Gradio, you'll create a powerful system capable of generating creative and coherent stories from simple prompts. Plus, you can follow along with a detailed YouTube video walkthrough that guides you through each step.
Technologies Used
- Python
- GPT-2
- Hugging Face Transformers
- Gradio
In this comprehensive guide, we will walk you through building your own AI Story Generator.
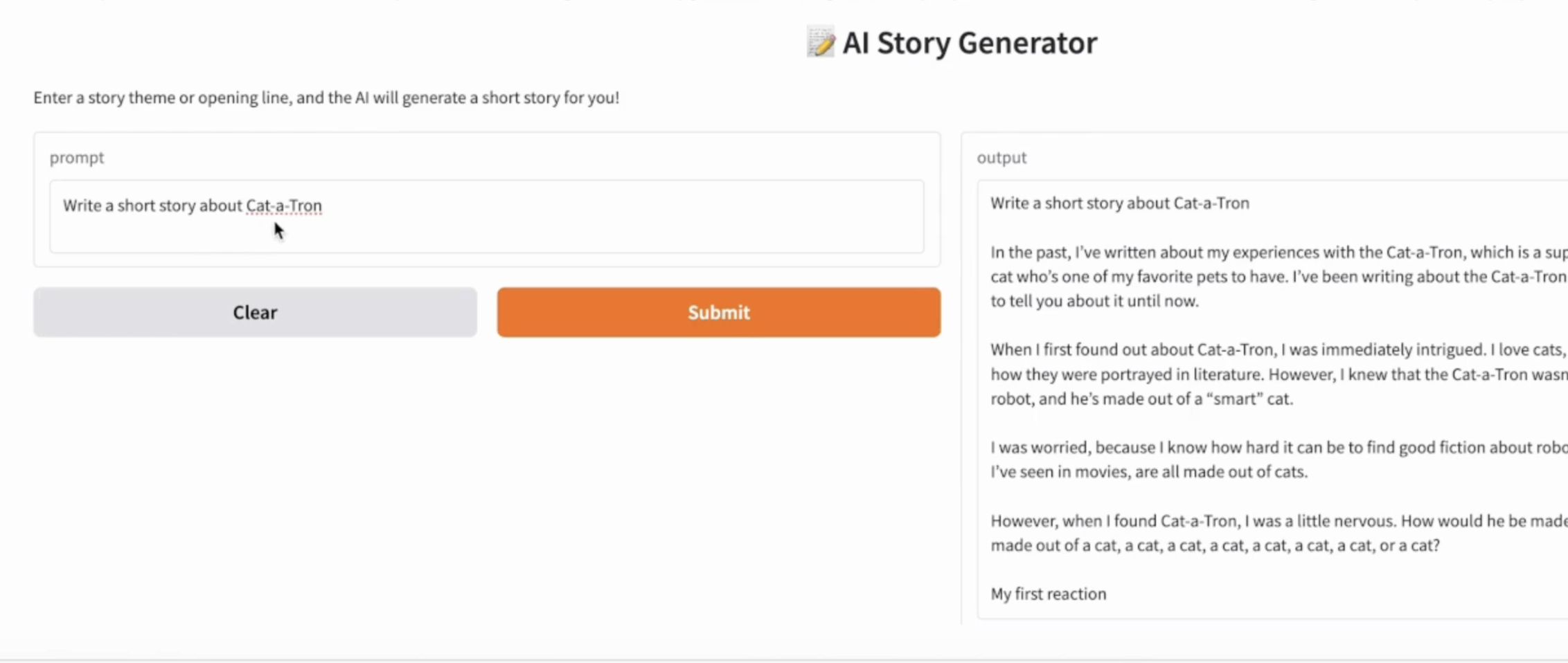
Why Build This AI?
The AI has several real-world uses, such as:
- Story Generation & Creative Writing
- Chatbots & Virtual Assistants
- Content Writing & Marketing
- Code Generation & Explanation
- Email & Report Automation
Let's Build It
Libraries Used
- Transformers: Hugging Face’s transformers library allows easy access to pre-trained models like GPT-2, GPT-3, etc.
- Gradio: Gradio provides an interactive interface to deploy and test the model quickly.
!pip install transformers gradio
Loading Pre-Trained Model:
Purpose: We load a pre-trained model (GPT-2 here) to generate text based on the input prompt.
Why: GPT-2 is widely used for text generation tasks due to its ability to create fluent, coherent text.
import gradio as gr
from transformers import pipeline
User Input and Text Generation
Purpose: The function generate_story
takes a prompt (e.g., "a cat") and generates a text story.
Why: We customize the input prompt to guide the model in generating text that's relevant to the user's query. The max_length
parameter limits the length of the generated story to avoid excessive run-time.
def generate_story(prompt):
story = story_generator(prompt,
max_length=300, # Generates longer stories
do_sample=True,
temperature=0.8, # Controls randomness
top_p=0.9,
top_k=50)
return story[0]["generated_text"]
Gradio Interface
Purpose: Gradio allows us to quickly create a simple web interface where users can input prompts and get text output.
Why: Gradio is easy to use and provides a quick solution for interacting with models in real time, without needing complex frontend development.
interface = gr.Interface(
fn=generate_story, # Function to generate stories
inputs=gr.Textbox(lines=2, placeholder="Enter a story theme or opening line..."),
outputs="text",
title="📝 AI Story Generator",
description="Enter a story theme or opening line, and the AI will generate a short story for you!",
)
interface.launch(share=True)